When you add or delete a child view controller, there is a number of things you need to do. For me it has always been too easy to forget what things I needed to do and in which order. So I decided to create this post as a little reminder for myself. All the quotes and code examples are from Apple’s “View Controller Programming Guide for iOS”.
Adding a Child View Controller
- Call the addChildViewController: method of your container view controller. This method tells UIKit that your container view controller is now managing the view of the child view controller.
- Add the child’s root view to your container’s view hierarchy. Always remember to set the size and position of the child’s frame as part of this process.
- Add any constraints for managing the size and position of the child’s root view.
- Call the didMoveToParentViewController: method of the child view controller.
Example:
1 2 3 4 5 6 |
- (void) displayContentController: (UIViewController*) content { [self addChildViewController:content]; content.view.frame = [self frameForContentController]; [self.view addSubview:self.currentClientView]; [content didMoveToParentViewController:self]; } |
Removing a Child View Controller
- Call the child’s willMoveToParentViewController: method with the value nil.
- Remove any constraints that you configured with the child’s root view.
- Remove the child’s root view from your container’s view hierarchy.
- Call the child’s removeFromParentViewController method to finalize the end of the parent-child relationship.
Example:
1 2 3 4 5 |
- (void) hideContentController: (UIViewController*) content { [content willMoveToParentViewController:nil]; [content.view removeFromSuperview]; [content removeFromParentViewController]; } |

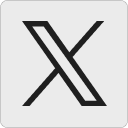



