Just yesterday I implemented social sharing in my new app called Sigma. Here is how I did it in two easy to follow steps.
First, I added Social.framework to my project and imported it to my view controller.
1 |
import Social |
Second, I wrote this code for Twitter (it’s in Swift 3.0):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
@IBAction func shareOnTwitter(_ sender: AnyObject) { if(SLComposeViewController.isAvailable(forServiceType: SLServiceTypeTwitter)){ let tweetSheet = SLComposeViewController(forServiceType: SLServiceTypeTwitter) tweetSheet?.setInitialText("initial text") tweetSheet?.add(image) tweetSheet?.add(URL.init(string: "http://yourlink.com")) tweetSheet?.completionHandler = {(result) in switch(result) { // This means the user cancelled without sending the Tweet case SLComposeViewControllerResult.cancelled: break; // This means the user hit 'Send' case SLComposeViewControllerResult.done: break; } } if (tweetSheet != nil){ present(tweetSheet!, animated: true, completion: nil) } }else{ let alert = UIAlertController(title: "Log in to Twitter", message: nil, preferredStyle: UIAlertControllerStyle.alert) let okAction = UIAlertAction(title: "OK", style: .default, handler: nil) alert.addAction(okAction) present(alert, animated: true, completion: nil) } } |
And this code for Facebook:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
@IBAction func shareOnFacebook(_ sender: AnyObject) { if(SLComposeViewController.isAvailable(forServiceType: SLServiceTypeFacebook)){ let fbSheet = SLComposeViewController(forServiceType: SLServiceTypeFacebook) fbSheet?.setInitialText("initial text") fbSheet?.add(image) fbSheet?.add(URL.init(string: "http://yourlink.com")) fbSheet?.completionHandler = {(result) in switch(result) { // This means the user cancelled without sending the Tweet case SLComposeViewControllerResult.cancelled: break; // This means the user hit 'Send' case SLComposeViewControllerResult.done: break; } } if (fbSheet != nil){ present(fbSheet!, animated: true, completion: nil) } }else{ let alert = UIAlertController(title: "Log in to Facebook", message: nil, preferredStyle: UIAlertControllerStyle.alert) let okAction = UIAlertAction(title: "OK", style: .default, handler: nil) alert.addAction(okAction) present(alert, animated: true, completion: nil) } } |
That’s it!

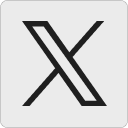



